/**
* 将字符串转换成二进制
* @param type $str
* @return type
*/
function StrToBin($str){
//1.列出每个字符
$arr = preg_split('/(?<!^)(?!$)/u', $str);
//2.unpack字符
foreach($arr as &$v){
$temp = unpack('H*', $v);
$v = base_convert($temp[1], 16, 2);
unset($temp);
}
return join(' ',$arr);
}
/**
* 将二进制转换成字符串
* @param type $str
* @return type
*/
function BinToStr($str){
$arr = explode(' ', $str);
foreach($arr as &$v){
$v = pack("H".strlen(base_convert($v, 2, 16)), base_convert($v, 2, 16));
}
return join('', $arr);
}
月度归档:2021年01月
php接口返回xml格式
在头部加上
header("Content-type: application/xml");
范例:
$res = $this->arrayToXml($data,$amount['count'],$page_count,$page); header("Content-type: text/xml"); echo $res;
//数组转XML
public function arrayToXml($data,$amount,$page_count,$page)
{
// var_dump($data);die;
$xml = "<response><code>0</code><msg>成功</msg><total_results>{$amount}</total_results><total_page>{$page_count}</total_page><current_page>{$page}</current_page><order_list>";
foreach ($data as $key=>$val)
{
$xml.= "<order><modified></modified><receiver_zip>-</receiver_zip><is_tax>false</is_tax><invoice_type></invoice_type><invoice_title></invoice_title><buyer_cod_fee>0.0</buyer_cod_fee><point_fee></point_fee><coupon_pay></coupon_pay><payments></payments>";
foreach($val['order'] as $k=>$v){
if (is_numeric($v)){
$xml.="<".$k.">".$v."</".$k.">";
}else{
$xml.="<".$k.">".$v."</".$k.">";
}
}
$xml.= "<itemlist>";
foreach($val['itemlist'] as $key=>$vv){
$xml.= "<item>";
foreach($vv as $kk=>$vs){
$xml.="<".$kk.">".$vs."</".$kk.">";
}
$xml.= "<shop_sku_id></shop_sku_id><sku_name></sku_name><oid></oid><amount></amount></item>";
}
$xml.= "</itemlist>";
$xml.= "</order>";
}
$xml.="</order_list></response>";
return trim($xml);
}
使用php://input接收数据流(还有多个参数的解决办法)
最近的工作中接触到一个手机上传图片到服务器的小程序,刚开始一直在想该用怎样的方式去接收数据,最后在网上看到大家都用php://input来接收。就去查了下php://input的官方介绍,确实可以,除了在enctype=”multipart/form-data”情况下,是无法接收到流的,其他情况下都可以。既然这个可以,我就在本地先写个测试文件
上传文件
<?php
//@file phpinput_post.php
$data=file_get_contents(‘btn.png’);
$http_entity_body = $data;
$http_entity_type = ‘application/x-www-form-urlencoded’;
$http_entity_length = strlen($http_entity_body);
$host = ‘127.0.0.1’;
$port = 80;
$path = ‘/image.php’;
$fp = fsockopen($host, $port, $error_no, $error_desc, 30);
if ($fp) {
fputs($fp, “POST {$path} HTTP/1.1\r\n”);
fputs($fp, “Host: {$host}\r\n”);
fputs($fp, “Content-Type: {$http_entity_type}\r\n”);
fputs($fp, “Content-Length: {$http_entity_length}\r\n”);
fputs($fp, “Connection: close\r\n\r\n”);
fputs($fp, $http_entity_body . “\r\n\r\n”);
while (!feof($fp)) {
$d .= fgets($fp, 4096);
}
fclose($fp);
echo $d;
}
?>
接收文件
运行test.php你就可以看到相应的结果,成功了,也在相应目录下看到了我刚才上传的文件。如果你做服务端,就只需要接收文件里面的php代码了。我上面模拟的上传就需要根据客户端来相应构造了,我后面也和客户端对接上了。所以是可以用的,还有就是客户端上传的时候可能会一些数据问题,因为我们在这个过程中出现了各种状况。
下文转自:http://www.0377joyous.com/archives/1135.html
今天公司要求用APP发送一个图片到PHP程序接收并保存起来,而且中间还需要很多参数!
以前没有做过APP和PHP交互,这次算是一个挑战吧(对一个没有人指导实习生来说)
1.APP发1.jpg,而且带有两个参数一个是假设是X和另外一个假设是Y
2.PHP负责接受X,Y和1.jpg,并且还要保存1.jpg到服务器
步骤:
1.PHP页面代码
$data = file_get_contents(‘php://input’);//这样可以获取到未经处理的原数据(保持发送的图片流不被破坏),在APP上使用X#Y#图片流使用http发送到PHP页面
//然后PHP页面进行数据处理和分割
2.数据处理
先分割数据流
$vars = explode(“#”,$data,3);//这样防止对图片流造成破坏只分割成三份即可
/省去若干代码/
$img = $vars[2];
$path = ‘/var/www/uploads/’;
$newfilename = time().”.jpg”;
$file = $path.$newfilename;
$handle = fopen($file, “w”);
if ($handle) {fwrite($handle,$img);
fclose($handle);
}
当PHP和C、C++、.net等应用程序进行开发的时候,应用程序使用POST方法传值过来PHP使用 $data=file_get_contents(‘php://input’),$data就是传过来的数据,而php://input不能用于 enctype=”multipart/form-data”。当需要给传值过来的程序 返回值的时候直接用echo返回就可以。
php解析xml转数组
php解析xml
数据并转换成数组
。使用simplexml_load_string()
转xml对象,json_encode()
把对象转成json,json_decode()
转成数组。
simplexml_load_file() 加载xml url
simplexml_load_string() 加载xml 字符串
- <?php
- $xml = ‘<?xml version=”1.0″ encoding=”utf-8″?>
- <res>
- <name>test</name>
- <age>10</age>
- <sex>man</sex>
- </res>’;
- $xml =simplexml_load_string($xml); //xml转object
- $xml= json_encode($xml); //objecct转json
- $xml=json_decode($xml,true); //json转array
- var_dump($xml);
php获取随机字符串的几种方法
方法一:shuffle函数(打乱数组)和mt_rand函数(生成随机数,比rand速度快四倍)
/** 获得随机字符串 @param $len 需要的长度 @param $special 是否需要特殊符号 @return string 返回随机字符串 */ function getRandomStr($len, $special=true){ $chars = array( "a", "b", "c", "d", "e", "f", "g", "h", "i", "j", "k", "l", "m", "n", "o", "p", "q", "r", "s", "t", "u", "v", "w", "x", "y", "z", "A", "B", "C", "D", "E", "F", "G", "H", "I", "J", "K", "L", "M", "N", "O", "P", "Q", "R", "S", "T", "U", "V", "W", "X", "Y", "Z", "0", "1", "2", "3", "4", "5", "6", "7", "8", "9" ); if($special){ $chars = array_merge($chars, array( "!", "@", "#", "$", "?", "|", "{", "/", ":", ";", "%", "^", "&", "*", "(", ")", "-", "_", "[", "]", "}", "<", ">", "~", "+", "=", ",", "." )); } $charsLen = count($chars) - 1; shuffle($chars); //打乱数组顺序 $str = ''; for($i=0; $i<$len; $i++){ $str .= $chars[mt_rand(0, $charsLen)]; //随机取出一位 } return $str; }
方法二、str_shuffle函数(打乱字符串顺序)和mt_rand函数
//取随机10位字符串 $strs="QWERTYUIOPASDFGHJKLZXCVBNM1234567890qwertyuiopasdfghjklzxcvbnm"; $name=substr(str_shuffle($strs),mt_rand(0,strlen($strs)-11),10); echo $name;
方法三、md5(),uniqid(),microtime()生成唯一的32位字符串
$uniqid = md5(uniqid(microtime(true),true)); //microtime(true) 返回系统当前时间戳的毫秒数
其他方法:
/**
* 方法一:获取随机字符串
* @param number $length 长度
* @param string $type 类型
* @param number $convert 转换大小写
* @return string 随机字符串
*/
function random($length = 6, $type = 'string', $convert = 0)
{
$config = array(
'number' => '1234567890',
'letter' => 'abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ',
'string' => 'abcdefghjkmnpqrstuvwxyzABCDEFGHJKMNPQRSTUVWXYZ23456789',
'all' => 'abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ1234567890'
);
if (!isset($config[$type]))
$type = 'string';
$string = $config[$type];
$code = '';
$strlen = strlen($string) - 1;
for ($i = 0; $i < $length; $i++) {
$code .= $string{mt_rand(0, $strlen)};
}
if (!empty($convert)) {
$code = ($convert > 0) ? strtoupper($code) : strtolower($code);
}
return $code;
}
/**
* 方法二:获取随机字符串
* @param int $randLength 长度
* @param int $addtime 是否加入当前时间戳
* @param int $includenumber 是否包含数字
* @return string
*/
function rand_str($randLength = 6, $addtime = 1, $includenumber = 0)
{
if ($includenumber) {
$chars = 'abcdefghijklmnopqrstuvwxyzABCDEFGHJKLMNPQEST123456789';
} else {
$chars = 'abcdefghijklmnopqrstuvwxyz';
}
$len = strlen($chars);
$randStr = '';
for ($i = 0; $i < $randLength; $i++) {
$randStr .= $chars[mt_rand(0, $len - 1)];
}
$tokenvalue = $randStr;
if ($addtime) {
$tokenvalue = $randStr . time();
}
return $tokenvalue;
}
CSS 背景位置 background-position属性
除了背景背景平铺外,CSS还提供了另一个强大的功能,即背景定位技术,能够精确控制背景在对象中的位置。
默认情况下,背景图像都是从元素 padding 区域的左上角开始出现的,但设计师往往希望背景能够出现在任何位置。通过 background-position属性,可以很轻松的控制背景图像在对象的背景区域中的起始显示位置。其语法格式为:
background-position: xpos ypos | x% y% | x y
CSS1中,background-position属性需要 2 个参数值,第一个值用于横坐标,第二个值用于纵坐标,默认值为 0% 0%,即背景图像的左上角与对象背景区域的左上角对齐。如果只提供一个值,则用于 x 轴方向,y 轴方向使用默认值 center,即垂直居中。
上述语法格式表明,背景图像有 3 种定位方式:
1)xpos ypos:表示使用预定义关键字定位,水平方向可选关键字有 left | center | right,垂直方向可选关键字有 top | center | bottom。
关键字定位,应用的是对齐规则,而不是坐标规则。xpos 为 left 表示图像的左边与对象的左边对齐,为 right 表示图像的右边和对象的右边对齐;ypos 为 top 表示图像的顶部和对象的顶部对齐,为 bottom 表示图像的底部和对象的底部对齐;xpos、ypos等于 center表示图像在水平和垂直方向的中心,和对象在水平和垂直方向的中心对齐,即。
依然使用前面的背景图案 bg.gif,其尺寸为 100px * 100px,对象的尺寸为 200px * 200px,背景图像的位置使用预定义关键字定位。如:
div {
width: 200px;
height: 200px;
border: 1px dashed #ccc;
background-repeat: no-repeat;
background-position: center center;
background-image: url(img/bg.gif);
}
上述定位,就表示背景图像的中心,与背景区域的中心对齐,即背景图像位于对象的中央位置。运行结果如图 4‑28 所示:

图4-28 背景图像关键字定位
2)x% y%:表示使用百分比定位,是将图像本身(x%, y%)的那个点,与背景区域的(x%, y%)的那个点重合。最终得到背景图像起始位置坐标的计算公式为:
x = (对象的宽度 – 图像的宽度) * x%;
y = (对象的高度 – 图像的高度) * y%;
还是上面的例子,改为百分比定位,要使背景图像居中,只需把背景图像的位置设置为50% 50% 即可。使用上面的公式,得到背景图像起始位置的坐标为:
x:(200px – 100px) * 50% = 100px * 50% = 50px
y:(200px – 100px) * 50% = 100px * 50% = 50px
得到的结果与上例相同,限于篇幅,就不再截图了。当然,百分比的值也可以是负值,计算公式依然不变。还是上面的例子,把百分比改为 -50% -50%。此时,得到背景图像起始位置的坐标为:
x:(200px – 100px) * (-50%) = 100px * (-50%) = -50px
y:(200px – 100px) * (-50%) = 100px * (-50%) = -50px
运行结果如图 4‑29 所示:

图4-29 背景图像百分比定位
从上图可以看出,此时的背景图像只显示了原本图像的 1/4,这是因为背景图像的起始位置向左、向上移动后,只有1/4 的图案落到了背景区域。由于背景被设置为不重复,所以,就只显示了原本图像的 1/4。
3)x y:表示使用长度值定位,是将背景图像的左上角,放置在对象的背景区域中(x, y)所指定的位置,即 x, y 定义的是背景图像的左上角,相对于背景区域左上角的偏移量。
偏移量长度可以是正值,也可以是负值。x 为正值表示向右偏移,负值表示向左偏移;y 为正值表示向下偏移,负值表示向上偏移。背景图像发生移动后,就有可能超出对象的背景区域。此时,超出的部分将不会显示,只会显示落入背景区域的部分。
如果把上面的例子改为长度值定位,要使背景图像居中,只需把背景图像的位置设置为50px 50px 即可。它就表示背景图像的左上角顶点,相对于对象背景区域的左上角顶点在 x轴右移 50px,在 y 轴下移 50px。得到的结果依然是背景图像位于对象的中央。
在CSS3中,background-position属性支持 4 个参数值,前两个值用于横坐标,后两个值用于纵坐标。这意味着我们可以相对于上左下右任意一个角落定位,而不是之前只能相对于左上角定位。可以用长度值、或百分比来指定背景图像的起始位置。
如果只提供一个值,则第二个值为center。如果提供两个值,第一个长度或百分比表示水平偏移,第二个长度或百分比表示垂直偏移。长度或百分比,表示背景图像的左上角相对于背景位置区域左上角的偏移。如,下面几个声明给出了背景的起点相对于背景区域左上角的偏移:
background-position: left 10px top 15px; /* 10px, 15px */
background-position: left top ; /* 0px, 0px */
background-position: 10px 15px; /* 10px, 15px */
background-position: left 15px; /* 0px, 15px */
background-position: 10px top ; /* 10px, 0px */
background-position: left top 15px; /* 0px, 15px */
background-position: left 10px top ; /* 10px, 0px */
原文:https://blog.csdn.net/ixygj197875/article/details/79333151
JS里的md5加密
https://cdn.bootcss.com/blueimp-md5/2.10.0/js/md5.js
源码:
/* * JavaScript MD5 * https://github.com/blueimp/JavaScript-MD5 * * Copyright 2011, Sebastian Tschan * https://blueimp.net * * Licensed under the MIT license: * https://opensource.org/licenses/MIT * * Based on * A JavaScript implementation of the RSA Data Security, Inc. MD5 Message * Digest Algorithm, as defined in RFC 1321. * Version 2.2 Copyright (C) Paul Johnston 1999 - 2009 * Other contributors: Greg Holt, Andrew Kepert, Ydnar, Lostinet * Distributed under the BSD License * See http://pajhome.org.uk/crypt/md5 for more info. */ /* global define */ ;(function ($) { 'use strict' /* * Add integers, wrapping at 2^32. This uses 16-bit operations internally * to work around bugs in some JS interpreters. */ function safeAdd (x, y) { var lsw = (x & 0xffff) + (y & 0xffff) var msw = (x >> 16) + (y >> 16) + (lsw >> 16) return (msw << 16) | (lsw & 0xffff) } /* * Bitwise rotate a 32-bit number to the left. */ function bitRotateLeft (num, cnt) { return (num << cnt) | (num >>> (32 - cnt)) } /* * These functions implement the four basic operations the algorithm uses. */ function md5cmn (q, a, b, x, s, t) { return safeAdd(bitRotateLeft(safeAdd(safeAdd(a, q), safeAdd(x, t)), s), b) } function md5ff (a, b, c, d, x, s, t) { return md5cmn((b & c) | (~b & d), a, b, x, s, t) } function md5gg (a, b, c, d, x, s, t) { return md5cmn((b & d) | (c & ~d), a, b, x, s, t) } function md5hh (a, b, c, d, x, s, t) { return md5cmn(b ^ c ^ d, a, b, x, s, t) } function md5ii (a, b, c, d, x, s, t) { return md5cmn(c ^ (b | ~d), a, b, x, s, t) } /* * Calculate the MD5 of an array of little-endian words, and a bit length. */ function binlMD5 (x, len) { /* append padding */ x[len >> 5] |= 0x80 << (len % 32) x[((len + 64) >>> 9 << 4) + 14] = len var i var olda var oldb var oldc var oldd var a = 1732584193 var b = -271733879 var c = -1732584194 var d = 271733878 for (i = 0; i < x.length; i += 16) { olda = a oldb = b oldc = c oldd = d a = md5ff(a, b, c, d, x[i], 7, -680876936) d = md5ff(d, a, b, c, x[i + 1], 12, -389564586) c = md5ff(c, d, a, b, x[i + 2], 17, 606105819) b = md5ff(b, c, d, a, x[i + 3], 22, -1044525330) a = md5ff(a, b, c, d, x[i + 4], 7, -176418897) d = md5ff(d, a, b, c, x[i + 5], 12, 1200080426) c = md5ff(c, d, a, b, x[i + 6], 17, -1473231341) b = md5ff(b, c, d, a, x[i + 7], 22, -45705983) a = md5ff(a, b, c, d, x[i + 8], 7, 1770035416) d = md5ff(d, a, b, c, x[i + 9], 12, -1958414417) c = md5ff(c, d, a, b, x[i + 10], 17, -42063) b = md5ff(b, c, d, a, x[i + 11], 22, -1990404162) a = md5ff(a, b, c, d, x[i + 12], 7, 1804603682) d = md5ff(d, a, b, c, x[i + 13], 12, -40341101) c = md5ff(c, d, a, b, x[i + 14], 17, -1502002290) b = md5ff(b, c, d, a, x[i + 15], 22, 1236535329) a = md5gg(a, b, c, d, x[i + 1], 5, -165796510) d = md5gg(d, a, b, c, x[i + 6], 9, -1069501632) c = md5gg(c, d, a, b, x[i + 11], 14, 643717713) b = md5gg(b, c, d, a, x[i], 20, -373897302) a = md5gg(a, b, c, d, x[i + 5], 5, -701558691) d = md5gg(d, a, b, c, x[i + 10], 9, 38016083) c = md5gg(c, d, a, b, x[i + 15], 14, -660478335) b = md5gg(b, c, d, a, x[i + 4], 20, -405537848) a = md5gg(a, b, c, d, x[i + 9], 5, 568446438) d = md5gg(d, a, b, c, x[i + 14], 9, -1019803690) c = md5gg(c, d, a, b, x[i + 3], 14, -187363961) b = md5gg(b, c, d, a, x[i + 8], 20, 1163531501) a = md5gg(a, b, c, d, x[i + 13], 5, -1444681467) d = md5gg(d, a, b, c, x[i + 2], 9, -51403784) c = md5gg(c, d, a, b, x[i + 7], 14, 1735328473) b = md5gg(b, c, d, a, x[i + 12], 20, -1926607734) a = md5hh(a, b, c, d, x[i + 5], 4, -378558) d = md5hh(d, a, b, c, x[i + 8], 11, -2022574463) c = md5hh(c, d, a, b, x[i + 11], 16, 1839030562) b = md5hh(b, c, d, a, x[i + 14], 23, -35309556) a = md5hh(a, b, c, d, x[i + 1], 4, -1530992060) d = md5hh(d, a, b, c, x[i + 4], 11, 1272893353) c = md5hh(c, d, a, b, x[i + 7], 16, -155497632) b = md5hh(b, c, d, a, x[i + 10], 23, -1094730640) a = md5hh(a, b, c, d, x[i + 13], 4, 681279174) d = md5hh(d, a, b, c, x[i], 11, -358537222) c = md5hh(c, d, a, b, x[i + 3], 16, -722521979) b = md5hh(b, c, d, a, x[i + 6], 23, 76029189) a = md5hh(a, b, c, d, x[i + 9], 4, -640364487) d = md5hh(d, a, b, c, x[i + 12], 11, -421815835) c = md5hh(c, d, a, b, x[i + 15], 16, 530742520) b = md5hh(b, c, d, a, x[i + 2], 23, -995338651) a = md5ii(a, b, c, d, x[i], 6, -198630844) d = md5ii(d, a, b, c, x[i + 7], 10, 1126891415) c = md5ii(c, d, a, b, x[i + 14], 15, -1416354905) b = md5ii(b, c, d, a, x[i + 5], 21, -57434055) a = md5ii(a, b, c, d, x[i + 12], 6, 1700485571) d = md5ii(d, a, b, c, x[i + 3], 10, -1894986606) c = md5ii(c, d, a, b, x[i + 10], 15, -1051523) b = md5ii(b, c, d, a, x[i + 1], 21, -2054922799) a = md5ii(a, b, c, d, x[i + 8], 6, 1873313359) d = md5ii(d, a, b, c, x[i + 15], 10, -30611744) c = md5ii(c, d, a, b, x[i + 6], 15, -1560198380) b = md5ii(b, c, d, a, x[i + 13], 21, 1309151649) a = md5ii(a, b, c, d, x[i + 4], 6, -145523070) d = md5ii(d, a, b, c, x[i + 11], 10, -1120210379) c = md5ii(c, d, a, b, x[i + 2], 15, 718787259) b = md5ii(b, c, d, a, x[i + 9], 21, -343485551) a = safeAdd(a, olda) b = safeAdd(b, oldb) c = safeAdd(c, oldc) d = safeAdd(d, oldd) } return [a, b, c, d] } /* * Convert an array of little-endian words to a string */ function binl2rstr (input) { var i var output = '' var length32 = input.length * 32 for (i = 0; i < length32; i += 8) { output += String.fromCharCode((input[i >> 5] >>> (i % 32)) & 0xff) } return output } /* * Convert a raw string to an array of little-endian words * Characters >255 have their high-byte silently ignored. */ function rstr2binl (input) { var i var output = [] output[(input.length >> 2) - 1] = undefined for (i = 0; i < output.length; i += 1) { output[i] = 0 } var length8 = input.length * 8 for (i = 0; i < length8; i += 8) { output[i >> 5] |= (input.charCodeAt(i / 8) & 0xff) << (i % 32) } return output } /* * Calculate the MD5 of a raw string */ function rstrMD5 (s) { return binl2rstr(binlMD5(rstr2binl(s), s.length * 8)) } /* * Calculate the HMAC-MD5, of a key and some data (raw strings) */ function rstrHMACMD5 (key, data) { var i var bkey = rstr2binl(key) var ipad = [] var opad = [] var hash ipad[15] = opad[15] = undefined if (bkey.length > 16) { bkey = binlMD5(bkey, key.length * 8) } for (i = 0; i < 16; i += 1) { ipad[i] = bkey[i] ^ 0x36363636 opad[i] = bkey[i] ^ 0x5c5c5c5c } hash = binlMD5(ipad.concat(rstr2binl(data)), 512 + data.length * 8) return binl2rstr(binlMD5(opad.concat(hash), 512 + 128)) } /* * Convert a raw string to a hex string */ function rstr2hex (input) { var hexTab = '0123456789abcdef' var output = '' var x var i for (i = 0; i < input.length; i += 1) { x = input.charCodeAt(i) output += hexTab.charAt((x >>> 4) & 0x0f) + hexTab.charAt(x & 0x0f) } return output } /* * Encode a string as utf-8 */ function str2rstrUTF8 (input) { return unescape(encodeURIComponent(input)) } /* * Take string arguments and return either raw or hex encoded strings */ function rawMD5 (s) { return rstrMD5(str2rstrUTF8(s)) } function hexMD5 (s) { return rstr2hex(rawMD5(s)) } function rawHMACMD5 (k, d) { return rstrHMACMD5(str2rstrUTF8(k), str2rstrUTF8(d)) } function hexHMACMD5 (k, d) { return rstr2hex(rawHMACMD5(k, d)) } function md5 (string, key, raw) { if (!key) { if (!raw) { return hexMD5(string) } return rawMD5(string) } if (!raw) { return hexHMACMD5(key, string) } return rawHMACMD5(key, string) } if (typeof define === 'function' && define.amd) { define(function () { return md5 }) } else if (typeof module === 'object' && module.exports) { module.exports = md5 } else { $.md5 = md5 } })(this)
小程序里使用,把最后的部分修改一下即可:
/* * JavaScript MD5 * https://github.com/blueimp/JavaScript-MD5 * * Copyright 2011, Sebastian Tschan * https://blueimp.net * * Licensed under the MIT license: * https://opensource.org/licenses/MIT * * Based on * A JavaScript implementation of the RSA Data Security, Inc. MD5 Message * Digest Algorithm, as defined in RFC 1321. * Version 2.2 Copyright (C) Paul Johnston 1999 - 2009 * Other contributors: Greg Holt, Andrew Kepert, Ydnar, Lostinet * Distributed under the BSD License * See http://pajhome.org.uk/crypt/md5 for more info. */ /* global define */ /* * Add integers, wrapping at 2^32. This uses 16-bit operations internally * to work around bugs in some JS interpreters. */ function safeAdd (x, y) { var lsw = (x & 0xffff) + (y & 0xffff) var msw = (x >> 16) + (y >> 16) + (lsw >> 16) return (msw << 16) | (lsw & 0xffff) } /* * Bitwise rotate a 32-bit number to the left. */ function bitRotateLeft (num, cnt) { return (num << cnt) | (num >>> (32 - cnt)) } /* * These functions implement the four basic operations the algorithm uses. */ function md5cmn (q, a, b, x, s, t) { return safeAdd(bitRotateLeft(safeAdd(safeAdd(a, q), safeAdd(x, t)), s), b) } function md5ff (a, b, c, d, x, s, t) { return md5cmn((b & c) | (~b & d), a, b, x, s, t) } function md5gg (a, b, c, d, x, s, t) { return md5cmn((b & d) | (c & ~d), a, b, x, s, t) } function md5hh (a, b, c, d, x, s, t) { return md5cmn(b ^ c ^ d, a, b, x, s, t) } function md5ii (a, b, c, d, x, s, t) { return md5cmn(c ^ (b | ~d), a, b, x, s, t) } /* * Calculate the MD5 of an array of little-endian words, and a bit length. */ function binlMD5 (x, len) { /* append padding */ x[len >> 5] |= 0x80 << (len % 32) x[((len + 64) >>> 9 << 4) + 14] = len var i var olda var oldb var oldc var oldd var a = 1732584193 var b = -271733879 var c = -1732584194 var d = 271733878 for (i = 0; i < x.length; i += 16) { olda = a oldb = b oldc = c oldd = d a = md5ff(a, b, c, d, x[i], 7, -680876936) d = md5ff(d, a, b, c, x[i + 1], 12, -389564586) c = md5ff(c, d, a, b, x[i + 2], 17, 606105819) b = md5ff(b, c, d, a, x[i + 3], 22, -1044525330) a = md5ff(a, b, c, d, x[i + 4], 7, -176418897) d = md5ff(d, a, b, c, x[i + 5], 12, 1200080426) c = md5ff(c, d, a, b, x[i + 6], 17, -1473231341) b = md5ff(b, c, d, a, x[i + 7], 22, -45705983) a = md5ff(a, b, c, d, x[i + 8], 7, 1770035416) d = md5ff(d, a, b, c, x[i + 9], 12, -1958414417) c = md5ff(c, d, a, b, x[i + 10], 17, -42063) b = md5ff(b, c, d, a, x[i + 11], 22, -1990404162) a = md5ff(a, b, c, d, x[i + 12], 7, 1804603682) d = md5ff(d, a, b, c, x[i + 13], 12, -40341101) c = md5ff(c, d, a, b, x[i + 14], 17, -1502002290) b = md5ff(b, c, d, a, x[i + 15], 22, 1236535329) a = md5gg(a, b, c, d, x[i + 1], 5, -165796510) d = md5gg(d, a, b, c, x[i + 6], 9, -1069501632) c = md5gg(c, d, a, b, x[i + 11], 14, 643717713) b = md5gg(b, c, d, a, x[i], 20, -373897302) a = md5gg(a, b, c, d, x[i + 5], 5, -701558691) d = md5gg(d, a, b, c, x[i + 10], 9, 38016083) c = md5gg(c, d, a, b, x[i + 15], 14, -660478335) b = md5gg(b, c, d, a, x[i + 4], 20, -405537848) a = md5gg(a, b, c, d, x[i + 9], 5, 568446438) d = md5gg(d, a, b, c, x[i + 14], 9, -1019803690) c = md5gg(c, d, a, b, x[i + 3], 14, -187363961) b = md5gg(b, c, d, a, x[i + 8], 20, 1163531501) a = md5gg(a, b, c, d, x[i + 13], 5, -1444681467) d = md5gg(d, a, b, c, x[i + 2], 9, -51403784) c = md5gg(c, d, a, b, x[i + 7], 14, 1735328473) b = md5gg(b, c, d, a, x[i + 12], 20, -1926607734) a = md5hh(a, b, c, d, x[i + 5], 4, -378558) d = md5hh(d, a, b, c, x[i + 8], 11, -2022574463) c = md5hh(c, d, a, b, x[i + 11], 16, 1839030562) b = md5hh(b, c, d, a, x[i + 14], 23, -35309556) a = md5hh(a, b, c, d, x[i + 1], 4, -1530992060) d = md5hh(d, a, b, c, x[i + 4], 11, 1272893353) c = md5hh(c, d, a, b, x[i + 7], 16, -155497632) b = md5hh(b, c, d, a, x[i + 10], 23, -1094730640) a = md5hh(a, b, c, d, x[i + 13], 4, 681279174) d = md5hh(d, a, b, c, x[i], 11, -358537222) c = md5hh(c, d, a, b, x[i + 3], 16, -722521979) b = md5hh(b, c, d, a, x[i + 6], 23, 76029189) a = md5hh(a, b, c, d, x[i + 9], 4, -640364487) d = md5hh(d, a, b, c, x[i + 12], 11, -421815835) c = md5hh(c, d, a, b, x[i + 15], 16, 530742520) b = md5hh(b, c, d, a, x[i + 2], 23, -995338651) a = md5ii(a, b, c, d, x[i], 6, -198630844) d = md5ii(d, a, b, c, x[i + 7], 10, 1126891415) c = md5ii(c, d, a, b, x[i + 14], 15, -1416354905) b = md5ii(b, c, d, a, x[i + 5], 21, -57434055) a = md5ii(a, b, c, d, x[i + 12], 6, 1700485571) d = md5ii(d, a, b, c, x[i + 3], 10, -1894986606) c = md5ii(c, d, a, b, x[i + 10], 15, -1051523) b = md5ii(b, c, d, a, x[i + 1], 21, -2054922799) a = md5ii(a, b, c, d, x[i + 8], 6, 1873313359) d = md5ii(d, a, b, c, x[i + 15], 10, -30611744) c = md5ii(c, d, a, b, x[i + 6], 15, -1560198380) b = md5ii(b, c, d, a, x[i + 13], 21, 1309151649) a = md5ii(a, b, c, d, x[i + 4], 6, -145523070) d = md5ii(d, a, b, c, x[i + 11], 10, -1120210379) c = md5ii(c, d, a, b, x[i + 2], 15, 718787259) b = md5ii(b, c, d, a, x[i + 9], 21, -343485551) a = safeAdd(a, olda) b = safeAdd(b, oldb) c = safeAdd(c, oldc) d = safeAdd(d, oldd) } return [a, b, c, d] } /* * Convert an array of little-endian words to a string */ function binl2rstr (input) { var i var output = '' var length32 = input.length * 32 for (i = 0; i < length32; i += 8) { output += String.fromCharCode((input[i >> 5] >>> (i % 32)) & 0xff) } return output } /* * Convert a raw string to an array of little-endian words * Characters >255 have their high-byte silently ignored. */ function rstr2binl (input) { var i var output = [] output[(input.length >> 2) - 1] = undefined for (i = 0; i < output.length; i += 1) { output[i] = 0 } var length8 = input.length * 8 for (i = 0; i < length8; i += 8) { output[i >> 5] |= (input.charCodeAt(i / 8) & 0xff) << (i % 32) } return output } /* * Calculate the MD5 of a raw string */ function rstrMD5 (s) { return binl2rstr(binlMD5(rstr2binl(s), s.length * 8)) } /* * Calculate the HMAC-MD5, of a key and some data (raw strings) */ function rstrHMACMD5 (key, data) { var i var bkey = rstr2binl(key) var ipad = [] var opad = [] var hash ipad[15] = opad[15] = undefined if (bkey.length > 16) { bkey = binlMD5(bkey, key.length * 8) } for (i = 0; i < 16; i += 1) { ipad[i] = bkey[i] ^ 0x36363636 opad[i] = bkey[i] ^ 0x5c5c5c5c } hash = binlMD5(ipad.concat(rstr2binl(data)), 512 + data.length * 8) return binl2rstr(binlMD5(opad.concat(hash), 512 + 128)) } /* * Convert a raw string to a hex string */ function rstr2hex (input) { var hexTab = '0123456789abcdef' var output = '' var x var i for (i = 0; i < input.length; i += 1) { x = input.charCodeAt(i) output += hexTab.charAt((x >>> 4) & 0x0f) + hexTab.charAt(x & 0x0f) } return output } /* * Encode a string as utf-8 */ function str2rstrUTF8 (input) { return unescape(encodeURIComponent(input)) } /* * Take string arguments and return either raw or hex encoded strings */ function rawMD5 (s) { return rstrMD5(str2rstrUTF8(s)) } function hexMD5 (s) { return rstr2hex(rawMD5(s)) } function rawHMACMD5 (k, d) { return rstrHMACMD5(str2rstrUTF8(k), str2rstrUTF8(d)) } function hexHMACMD5 (k, d) { return rstr2hex(rawHMACMD5(k, d)) } function md5 (string, key, raw) { if (!key) { if (!raw) { return hexMD5(string) } return rawMD5(string) } if (!raw) { return hexHMACMD5(key, string) } return rawHMACMD5(key, string) } module.exports = { md5: md5 }
java 集合按照ASCII码从小到大(顺序)排序
import java.util.SortedMap;
import java.util.TreeMap;
public class TestTreeMap {
public static void main(String[] args) {
SortedMap <String, Object> params = new TreeMap <>();
params.put("ZKK", "15");
params.put("AT", "1525524700740");
params.put("NONCESTR", "1522115166482");
params.put("PASSWORD", "123456");
params.put("PHONEID", "android");
params.put("PHONEIP", "PHONEIP");
params.put("USERNAME", "13800000001");
params.put("APPID", "A80C1A90A90C4260B52CB8FE559F70BD");
System.out.println(params);
}
}
CSS实现垂直居中的几种方法
======
目录
正文回到顶部
单行文本的居中
1.文字水平居中
1 <div class='box' style="text-align: center;">hello world</div>
2.文本垂直水平居中
1 <div class="box2" style="width:150px;height:100px;line-height: 100px;">文本垂直水平居中</div>
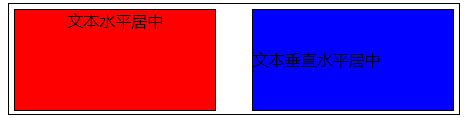
二、多行文本的垂直居中
1.使用display:flex实现
flex布局会让容器内的元素得到垂直水平居中

1 <!DOCTYPE html> 2 <html> 3 <head> 4 <meta charset="utf-8"> 5 <title>登陆</title> 6 <style type="text/css"> 7 html{width: 100%;height: 100%;} /*整个页面的居中*/ 8 body{ 9 width: 100%; 10 height: 100%; 11 display: flex; /*flex弹性布局*/ 12 justify-content: center; 13 align-items: center; 14 } 15 #login{ 16 width: 300px; 17 height: 300px; 18 border: 1px black solid; 19 display: flex; 20 flex-direction: column; /*元素的排列方向为垂直*/ 21 justify-content: center; /*水平居中对齐*/ 22 align-items: center; /*垂直居中对齐*/ 23 } 24 </style> 25 </head> 26 <body> 27 <div id="login"> 28 <h1>登陆</h1> 29 <input type="text"><br> 30 <input type="password"><br> 31 <button>确定</button> 32 </div> 33 </body> 34 </html>

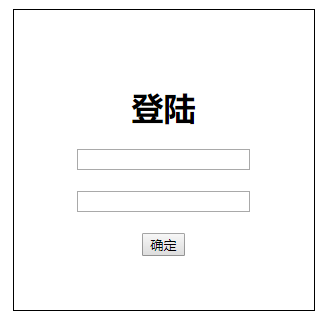
2.使用display:-webkit-box实现

1 body{ 2 width: 100%; 3 height: 100%; 4 display: -webkit-box; /*flex弹性布局*/ 5 -webkit-box-align: center; 6 -webkit-box-pack: center; 7 }

display:flex和display:box都可用于弹性布局实现水平垂直居中,不同的是display:box是2009年的命名,已经过时,用的时候需要加上前缀;display:flex是2012年之后的命名
3.使用绝对定位和负边距
CSS代码:

<style> .box{ width: 150px; height: 150px; background:blue; position: relative; } p{ width: 50px; height: 50px; background:red; position: absolute; left:50%; top:50%; margin-left:-25px; margin-top: -25px; display: flex; align-items: center; justify-content: center; } </style>

HTML代码:
1 <div class="box"><p>A</p></div>
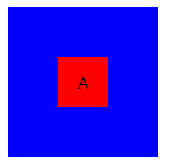
4.使用transform:translate定位

1 <style> 2 *{padding: 0;margin: 0;} /*解决容器内元素.div是p元素产生的居中不完整*/ 3 .box{ 4 margin: 20px auto; 5 width: 150px;height: 150px; 6 background:blue; 7 position: relative; 8 text-align: center; 9 } 10 .box .div1{ 11 position: absolute; 12 top:50%; 13 left:50%; 14 width:100%; 15 transform:translate(-50%,-50%); 16 text-align: center; 17 background: red 18 } 19 </style>

说明:/*一般情况下子元素不能是p元素,否则非完全居中,P元素自带有padding距离*/,.div1如果必须是p元素则必须加上*{margin:0;padding:0;};进行初始化,
5.绝对定位和0

1 .box p{ 2 width:50%; 3 height: 50%; 4 overflow: auto; 5 position: absolute; 6 background:red; 7 margin: auto; 8 top:0; 9 bottom:0; 10 left:0; 11 right:0; 12 }

6.通过display:table-cell

1 .box{ 2 width: 150px;height: 150px; 3 background:blue; 4 position: relative; 5 text-align: center; 6 display: table-cell; 7 vertical-align: middle; 8 }

缺点:对容器.box的子元素的设置宽高会造成失效。
微信小程序wx.request使用post方式传参入坑
急急忙忙尝试了2天,发现问题始终解决不掉
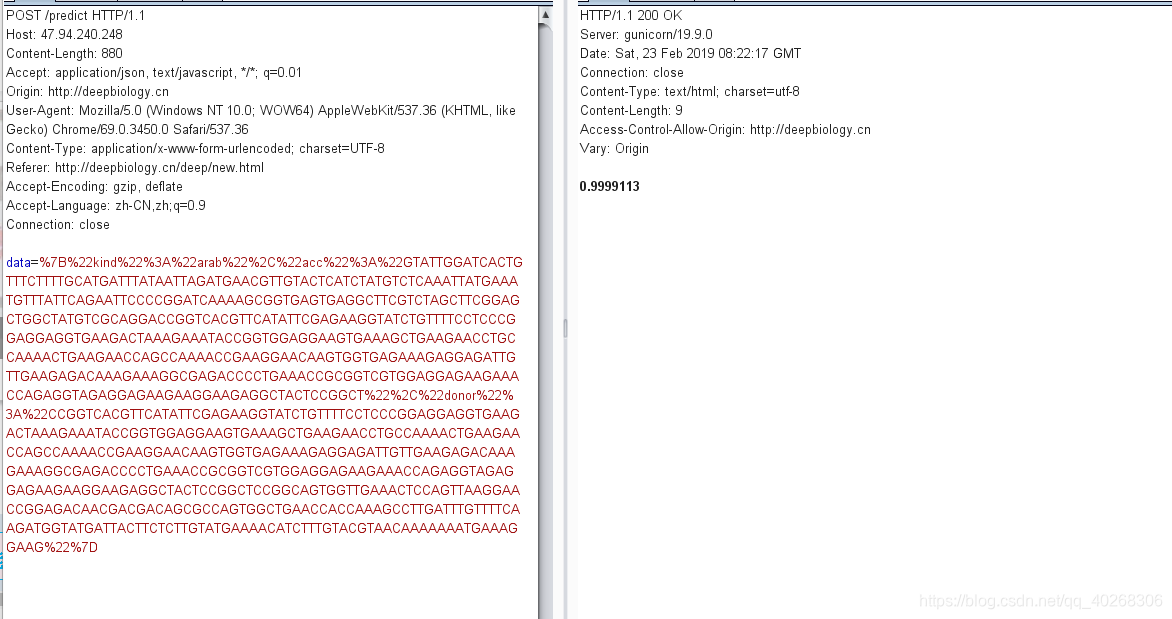
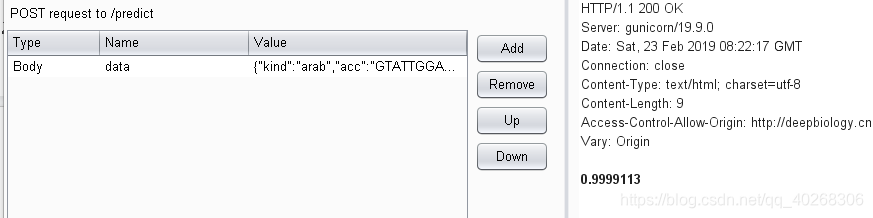
问题:通过微信小程序实现post
(后端是python flask
flask的post函数)
解决方案:凭我的经验猜测 只传输一个值 data ,然后里面包含字典形式的三个元素
{“kind”:””,
“acc”:””,
“donor”:””}
为了转换在小程序上post我费了些时间
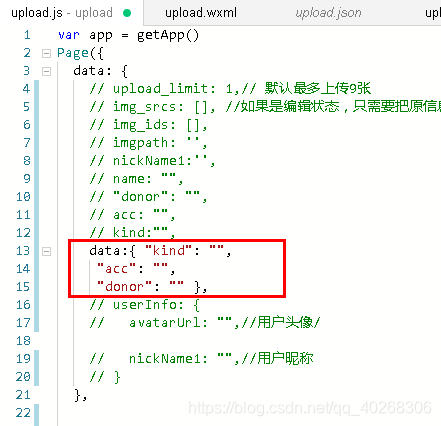
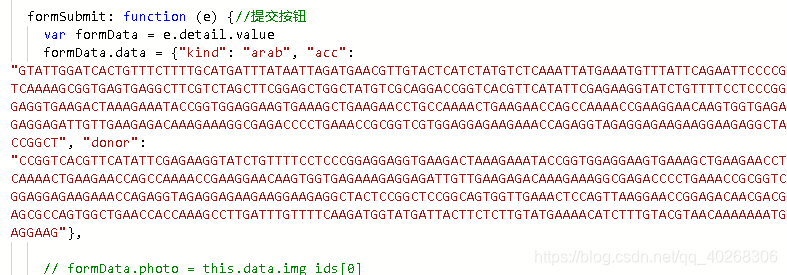
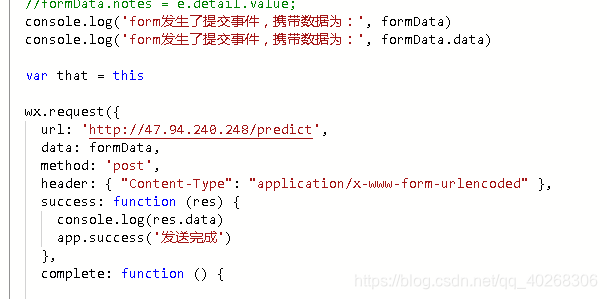
我感觉上述没有问题,但是不知道为什么就是通不过一直 500
下面是微信小程序的客户端post的
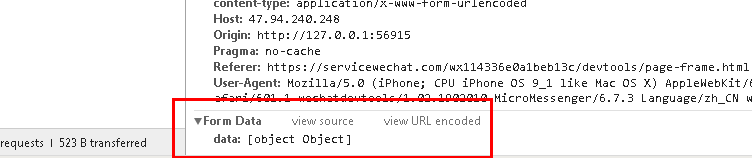
结果:
我猜测还是我post的数据格式不对,
相应200的 正确格式
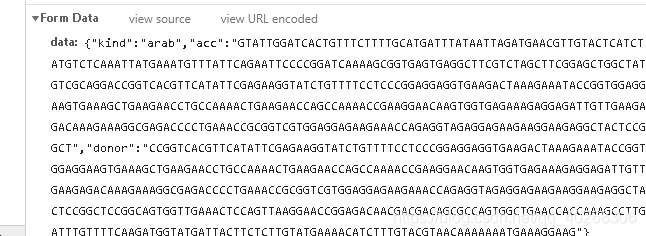
最终解决方案:
采用
在前后端交互的过程中难免会出现需要我们将字符串转成json的时候。
json.stringify()方法是将一个JavaScript值(对象或者数组)转换为一个 JSON字符串
json.parse() 方法将数据转换为 JavaScript 对象( 将字符串转成json对象。 )
强烈安利JavaScript 教程
后记:
再传post值的过程中
应先抓包分析一下对应的 post为和值,或者直接利用开发者工具箱直接network 拉到最后直接分析
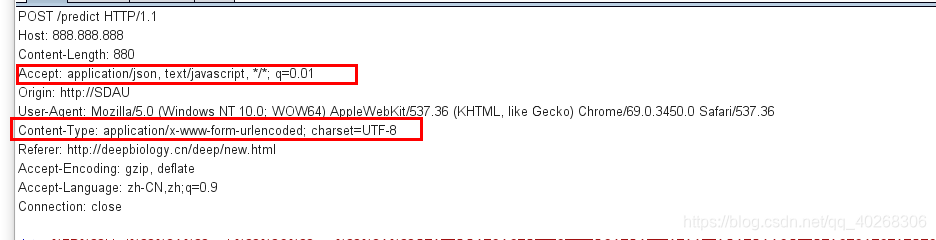
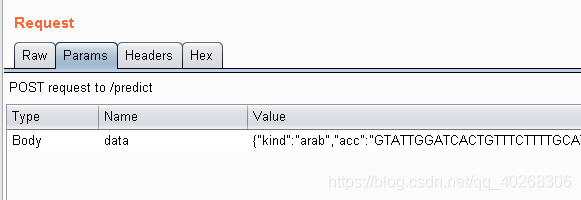
通过content-type 得到传参方式 最下方仅为post的数据,我没农商
一般来说传参方式分为
application/json multipart/form-data application/x-www-form-urlencoded
为什么会有这么多传参方式,就是因为他的编码方式,协议规定 POST 提交的数据必须放在消息主体(entity-body)中,但协议并没有规定数据必须使用什么编码方式。 数据发送出去,还要服务端解析成功才有意义。一般服务端语言如 php、python 等,以及它们的 framework,都内置了自动解析常见数据格式的功能。
服务端通常是根据请求头(headers)中的 Content-Type 字段来获知请求中的消息主体是用何种方式编码,再对主体进行解析。
所以说到 POST 提交数据方案,包含了 Content-Type 和消息主体编码方式两部分。
原文:https://blog.csdn.net/qq_40268306/article/details/87893789